Clever's Data Model
Here, we'll walk you through how records are related in our data model. You can query for related records using relative links returned in the response unless otherwise noted. For example, /schools/{id}/sections will return all sections whose primary school is {id}
The diagram for each record type lists associated records. Associated records connected with a solid line have their IDs listed in the record object; records connected with a dotted line must be accessed through a relative link or other endpoint.
Schema
Below each object, we've added a sample record with available fields - we've sorted the fields for clarity, but they may be returned in any order. Please note the table as well, which outlines which fields are (and are not) guaranteed.
Field formats
- Object ID: All Clever IDs take the ObjectID format. We guarantee that ObjectIDs are globally unique across districts and user types.
- String: Clever's
strings
have no maximum length - if you have to set maximum character lengths for fields, we recommend setting the max to 255 characters for safety. - Date: We normalize dates in Clever to YYYY-MM-DD: "2015-08-25"
- Timestamp: Timestamps are expressed in this format: "2015-08-25T00:00:00.000Z"
- Boolean: Booleans have a value of either "Y" or "N"
Links
Relative links are one way to discover and navigate to related data.
Let's examine the tail end of the JSON response to /me while using a student instant login token. Here's an example links node:
"links":
[
{ "rel":"self","uri":"/me" },
{ "rel":"canonical","uri":"/v2.1/students/4fee004cca2e43cf2700028b" },
{ "rel":"district","uri":"/v2.1/districts/4feecb637de9810111e000002" }
]
Each relative link is structured:
rel
- a string containing a keyword describing why you might be interested in the uri part
uri
- a string containing the path component of a Clever API request
The rel keyword you're most interested in while handling this step is canonical. This is a fancy way of saying that the primary way to retrieve information about the object described in your API request is by requesting the path in the uri key.
To use that uri, you would attach the path component to https://api.clever.com
. In the above canonical example, that would be https://api.clever.com/v2.1/students/4fee004cca2e43cf2700028b
. This path will be accessible using the same instant login token or the appropriate district bearer token.
We strongly recommend following relative links to access related pieces of data. Constructing URLs by hand can be prone to errors and by following relative links, you'll be more resilient to access pattern changes in the Clever API.
Districts
Clever has a district-centric data model – our top level object is the district. Districts contain schools, terms, courses, sections, teachers, students, school_admins, and district_admins, and contacts.
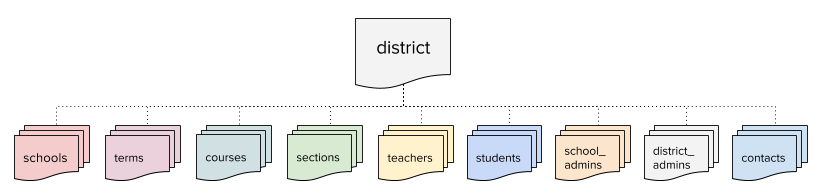
District object
{
"data": {
"id": "", // ObjectID: Globally unique and stable ID for district created by Clever,
"name": "", // String: Name provided by district
"state": "", // String: District's sync status
"last_sync": "", // Timestamp: Last successful sync of district's data
"launch_date": "", // Date: When district's users will have access to Instant Login
"mdr_number": "", // String: MDR number
"nces_id": "", //Federal NCES id for district
"error": "", // String: Error state
"sis_type": "", // String: Type of SIS district data originates from
"pause_start": "", // Date: When district's data will be paused
"pause_end": "", // Date: When district's data will be unpaused
"district_contact": {
"district": "", //Object ID: Globally unique and stable ID for district
"email": "", //String: Contact's email address
"name": {
"first": "", //String: Contact's first name
"last": "", //String: Contact's last name
},
"title": "", //String: Contact's title
"id": "" //ObjectID: Globally unique and stable ID for contact
},
"portal_url": "", //String: URL where users log in to Clever
"login_methods": {
"", // List of supported login types
""
},
},
"uri": "/v2.1/districts/id/"
}
Guaranteed fields
id
name
state
- Possible values: ["running", "pending", "error", "paused", "success", ""] Pending means there is an error that the district can fix; Error means there is an error that Clever can fix. Paused means that the district's sync is paused to reflect last year's data.last_sync
- Populated as long as the district has synced datalaunch_date
goals_enabled
portal_url
login_methods
Optional fields
mdr_number
nces_id
error
- User-facing string describing a district error state. Typically useful only for district administrators. Should not be used to programmatically determine district status.sis_type
- Indicates the type of SIS this district's data originates from. "sftp" is returned when district data is synced via SFTP or CSV upload. Other string values vary.pause_start
pause_end
district_contact
Related objects
The district object itself has only the name
, Clever id
, mdr_number
, and nces_id
(if assigned) of the district in question. You'll need to use separate endpoints to access the district's data. For example, since each token will give you access to only a single district, you should use https://api.clever.com/v2.1/schools
. While courses, terms, contacts, school admins, and district admins are associated with a district, there is no relative link returned for these users on the district endpoint.
Instead, you can use:
- Terms
- /terms
- Courses
- /courses
- Contacts
- /contacts
- District Admins
- /district_admins
- School Admins
- /school_admins
Schools
While schools are not the highest-level object in Clever's data model, every student, teacher, and section must be associated with at least one school. School admin records can be associated with multiple schools!
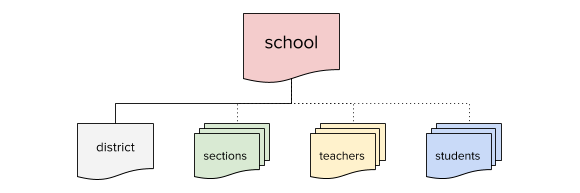
School objects
{
"data": {
"id": "", // ObjectID: Globally unique and stable ID for school created by Clever
"district": "", // ObjectID: Globally unique and stable ID for school's district
"name": "", // String: Name provided by district
"created": "", // Timestamp: Resource creation date
"last_modified": "", // Timestamp: Last time resource was updated.
"sis_id": "", // String: Internal school identifier from SIS
"school_number": "", // String: School identifier used by district or county
"state_id": "", // String: State school identifier
"nces_id": "", // String: School NCES ID
"mdr_number": "", // String: School MDR number
"low_grade": "", // String: School's beginning grade level
"high_grade": "", // String: School's exit grade level
"location": {
"zip": "", // String: School's zip code
"address": "", // String: School's street address
"city": "", // String: School's city
"state": "" // String: School's state
},
"phone": "", // String School's phone number
"principal": {
"email": "", // String: School's principal's email address
"name": "" // String: School's principal's name
},
"ext": {
"": "" // String: Extension field names and values are defined by the district
},
},
"uri": "/v2.1/schools/id"
}
Guaranteed fields
id
district
name
created
last_modified
- Initializes to created datesis_id
school_number
Optional fields
state_id
nces_id
mdr_number
low_grade
- Possible values [ "1", … ,"13", "PreKindergarten", "TransitionalKindergarten", "Kindergarten", "InfantToddler", "Preschool", "PostGraduate", "Ungraded", "Other", ""]high_grade
- Possible values [ "1", … ,"13", "PreKindergarten", "TransitionalKindergarten", "Kindergarten", "InfantToddler", "Preschool", "PostGraduate", "Ungraded", "Other", ""]principal
location
phone
ext
Related objects
To access the sections, teachers, or students associated with a school, use these endpoints:
Terms
Terms are an optional data type - these are only created if a district sends us term information.
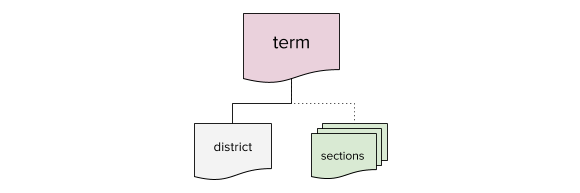
Term objects
{
"data": {
"id": "", // ObjectID: Globally unique and stable ID for the term
"district": "", // ObjectID: Globally unique and stable ID for the term's district
"name": "", // String: Name provided by district
"start_date": "", // Date: Term's start date
"end_date": "" // Date: Term's end date
},
"uri": "/v2.1/terms/id"
}
Guaranteed fields
id
district
- At least one of the optional fields listed below
Optional fields
name
start_date
end_date
Related objects
To retrieve a list of the section objects associated with a term, use /terms/{id}/sections.
Courses
Courses are an optional data type - courses are only created if a district chooses to sync course information to Clever.
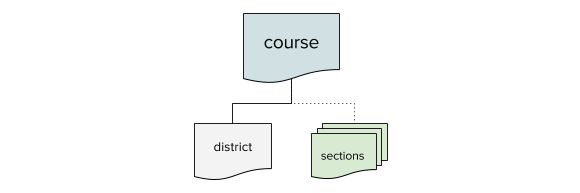
Course objects
{
"data": {
"id": "", // ObjectID: Globally unique and stable ID for the course
"district": "", // ObjectID: Globally unique and stable ID for the course's district
"name": "", // String: name provided by district
"number": "" // String: course number provided by district
},
"uri": "/v2.1/courses/id"
}
Guaranteed fields
id
district
- At least one of the optional fields listed below
Optional fields
name
number
Related objects
To retrieve a list of the section objects associated with a course, use /courses/{id}/sections.
Sections
Sections (analagous to classes) tie teachers and students together.
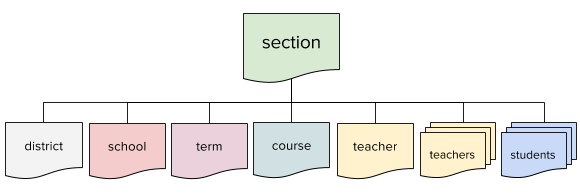
Section objects
{
"data": {
"id": "", // Globally unique and stable ID for the section
"district": "", // ObjectID: Globally unique and stable ID for section's district
"school": "", // ObjectID: Globally unique and stable ID for section's school
"created": "", // Timestamp: Resource initialization date
"last_modified": "", // Timestamp: Last time resource was updated
"name": "", // String: Name provided by district or generated by Clever
"sis_id": "", // String: Internal section identifier from SIS
"subject": "", // String: Subject associated with section
"students": [ // List of ObjectIDs: List of IDs of students enrolled in section
"",
""
],
"teacher": "", // ObjectID: Globally unique and stable ID for section's primary teacher
"teachers": [ // List of ObjectIDs: List of IDs of teachers assocatedw with section
"",
""
],
"grade": "", // String: Grade associated with section
"course": "", // ObjectID: Globally unique and stable ID for section's course
"term_id": "", // ObjectID: Globally unique and stable ID for section's term
"section_number": "", // String: Section number set by school or district
"period": "" // String: Bell schedule information for the section
},
"ext": {
"": "" // String: Extension field names and values are defined by the district
},
"uri": "/v2.1/sections/id"
}
Guaranteed fields
id
district
school
created
last_modified
name
- Clever generates a section name by concatenating "course_name" "- teacher_last_name" and "- period", e.g. Algebra - Smith - Period 3. If course name is not present, the section name from the source system is used.sis_id
subject
- Possible values: ["english/language arts","math","science","social studies","language","homeroom/advisory", "interventions/online learning","technology and engineering","PE and health","arts and music","other",""]students
teacher
teachers
Optional fields
grade
- Possible values [ "1", … ,"13", "PreKindergarten", "TransitionalKindergarten", "Kindergarten", "InfantToddler", "Preschool", "PostGraduate", "Ungraded", "Other", ""]course
term
section_number
period
ext
Related objects
Each section has a primary school and contains students and teacher objects. But be careful, the students and teachers may have a different primary school than the section. For example, an elementary student may be enrolled in a middle school math class.
You'll notice that the section has a teacher
field as well as a list of teachers
. If a section has more than one teacher, additional teachers are listed in the teachers
array. We recommend importing all teachers, not just the primary teacher.
Students
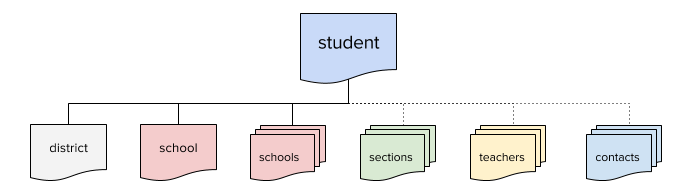
Student objects
{
"data": {
"id": "", // ObjectID: Globally unique and stable ID for student
"district": "", // ObjectID: Globally unique and stable ID for students district
"school": "", // ObjectID: Globally unique and stable ID for student's primary school
"schools": [ // List of ObjectIDs: List of IDs for schools student is associated with
"",
""
],
"created": "", // Timestamp: Resource initialization date
"last_modified": "", // Timestamp: Last time resource was modified
"sis_id": "", // String: Internal student identifier from SIS
"name": {
"last": "", // String: Last name provided by district
"middle": "", // String: middle name provided by district
"first": "" // String: First name provided by district
},
"dob": "", // String: Student birthdate
"email": "", // String: Email address provided by district
"student_number": "", // String: Student number provided by district
"state_id": "", // String: State student identifier
"credentials": {
"district_username": "" // String: District-specified student username
},
"gender": "", // String: Student gender
"race": "", // String: Student race
"hispanic_ethnicity": "", // Boolean: Student's hispanic/latino ethnicity
"location": {
"state": "", // String: Student state
"zip": "", // String: Student zip
"address": "", // String: Student street address
"city": "", // String: Student city
"lat": "", // String: Student address latitude (Deprecated)
"lon": "" // String: Student address longitude (Deprecated)
},
"graduation_year": "", // String: Graduation year provided by district
"grade": "", // String: Student grade
"home_language": "", // String: Language spoken in the home setting
"enrollments": { //For student school enrollment tracking
"school": "", // ObjectID: Globally unique and stable ID for the school
"start_date": "", // Date: When student first enrolled in the school in Clever
"end_date": "" // Date: When student's enrollment in the school ended
},
"ext": {
"": "" // String: Extension field names and values are defined by the district
},
},
"uri": "/v2.1/students/id"
}
Guaranteed fields
id
district
school
schools
created
last_modified
sis_id
name.first
name.last
enrollments
Optional fields
name.middle
email
student_number
state_id
credentials.district_username
gender
- Possible values: ["M", "F"]race
- Possible values: ["Caucasian", "Asian", "Black or African American", "American Indian", "Hawaiian or Other Pacific Islander", "Two or More Races","Unknown", ""]hispanic_ethnicity
location
graduation_year
grade
home_language
ext
- enrollments
Related objects
Teachers
As you would expect, users with type teachers are typically instructional staff associated with sections. But you should also be aware that districts may include non-instructional staff (e.g. aides, principals, secretaries) as teachers and will likely not be associated with sections.
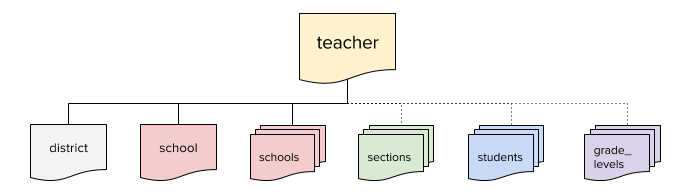
Teacher objects
{
"data": {
"id": "", // ObjectID: Globally unique and stable ID for teacher
"district": "", // ObjectID: Globally unique and stable ID for teacher's district
"school": "", // ObjectID: Globally unique and stable ID for teacher's primary school
"schools": [ // List of ObjectIDs: List of IDs for all schools teacher is associated with
"",
""
],
"created": "", // Timestamp: Resource initialization date
"last_modified": "", // Timestamp: Last time resource was updated
"sis_id": "", // String: Internal teacher identifier from SIS
"email": "", // String: Email address provided by district
"teacher_number": "", // String: Teacher number provided by district
"name": {
"first": "", // String: First name provided by district
"last": "", // String: Last name provided by district
"middle": "" // String: Middle name provided by district
},
"state_id": "", // String: State teacher identifer
"title": "", // String: Title provided by district
"credentials": {
"district_username": "" // String: District-specified username for teacher
},
},
"ext": {
"": "" // String: Extension field names and values are defined by the district
},
"uri": "/v2.1/teachers/id"
}
Guaranteed fields
id
district
school
schools
created
last_modified
sis_id
name.first
name.last
Optional fields
name.middle
email
teacher_number
state_id
title
credentials.district_username
Related objects
- /teachers/{id}/schools
- /teachers/{id}/sections
- /teachers/{id}/sections
- /teachers/{id}/students
- teachers/{id}/grade_levels
School Administrators
School administrators are not synced from the SIS; they are created via CSV upload to Clever. These users may be associated with multiple schools.
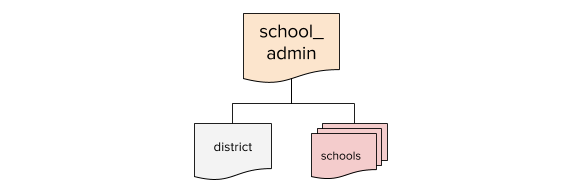
School administrator objects
{
"data": {
"email": "", // String: Admin's email address
"name": {
"first": "", // String: First name provided by district
"last": "" // String: Last name provided by district
},
"title": "", // String: Title provided by district
"department": "", // String: Department provided by district
"district": "", // ObjectID: Globally unique and stable ID for admin's district
"schools": [ // List of ObjectIDs: List of schools associated with admin
"",
""
],
"staff_id": "", // String: Identifier provied by district
"id": "" // ObjectID: Globally unique and stable ID for admin
},
"ext": {
"": "" // String: Extension field names and values are defined by the district
},
"uri": "/v2.1/school_admins/id"
}
Guaranteed fields
id
email
district
schools
created
last_modified
staff_id
name.first
name.last
Optional fields
department
title
Related objects
District Administrators
District administrators are users who have the ability to manage the data syncing into Clever and share it with applications.
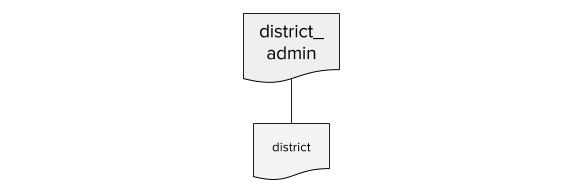
District administrator objects
{
"id": "", // ObjectID: Globally unique and stable ID for district admin
"district": "", // ObjectID: Globally unique and stable ID for admin's district
"name": {
"first": "", // String: First name provided by district
"last": "" // String: Last name provided by district
},
"email": "", // String: Admin's email address
"title": "" // String: Title provided by district
}
Guaranteed fields
id
district
name
email
Optional fields
title
Related objects
There are no relative links on district admins.
Student Contacts
Contacts are not directly associated with schools, only to the district and to students.
Important caveats around using student contact data
While we offer student contact information, it is not normalized in the same way that other data is in Clever. Please note:
- The quality and formatting of student contact data can vary between SISs
- Contacts without
sis_id
populated are not stable - if any data on the contact changes (such as name, email, or phone number), the contact will be deleted and a new one will be created with a new Clever ID.For more information, check out our page on Contacts
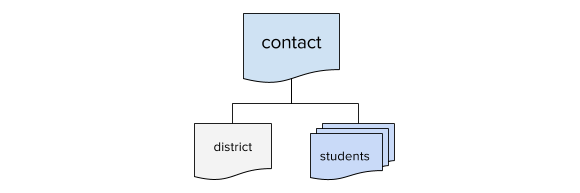
Contact objects
{
"data": {
"id": "", // ObjectID: Globally unique ID for contact
"district": "", // ObjectID: Globally unique and stable ID for contact district
"name": "", // String: Name provided by district
"type": "", // String: Contact type
"students": [ // List of ObjectIDs: List of students associated with contact
"",
""
],
"sis_id": "", // String: Internal identifier for contact assigned by SIS
"email": "", // String: Email address
"phone": "", // String: Contact phone number
"phone_type": "", // String: Phone number type
"relationship": "" // String: Contact relationship
},
"uri": "/v2.1/contacts/id"
}
Guaranteed fields
Field Name | Format | Description |
---|---|---|
id | ObjectID | Globally unique and stable id for contact. |
district | ObjectID | Globally unique and stable ID for contact district |
name | String | Full name provided by the district. |
type | String | Type of contact. Possible values: Primary , Secondary , Parent/Guardian , Emergency , Family , Other |
students | Array of ObjectIDs | List of student IDs associated with contact. |
Optional fields
Field Name | Format | Description |
---|---|---|
sis_id | String | Internal identifier for contact assigned by SIS. |
email | String | Contact email address. |
phone | String | Contact phone number. |
phone_type | String | Type of phone number. Possible values: Cell , Home , Work , Other . |
relationship | String | Relationship to student. Possible values: Parent , Grandparent , Self , Aunt/Uncle , Sibling , Other . |
Related objects
Updated over 3 years ago